What is it?
SimpleCV is an open source framework for building computer vision applications. With it, you get access to several high-powered computer vision libraries such as OpenCV – without having to first learn about bit depths, file formats, color spaces, buffer management, eigenvalues, or matrix versus bitmap storage. This is computer vision made easy.
Getting Started
from SimpleCV import Camera
# Initialize the camera
cam = Camera()
# Loop to continuously get images
while True:
# Get Image from camera
img = cam.getImage()
# Make image black and white
img = img.binarize()
# Draw the text "Hello World" on image
img.drawText("Hello World!")
# Show the image
img.show()
This example shows a "Hello World" program that uses SimpleCV. In this example we first connect to a USB web camera. We then capture images from the web cam, apply a binary threshold that turns our image black and white, and then draws some text. The program then prints the results to a display.
Examples
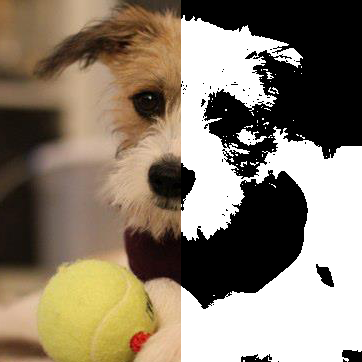
This image shows the SimpleCV threshold function. The threshold method sets each pixel in an image to black or white depending on its brightness. Example
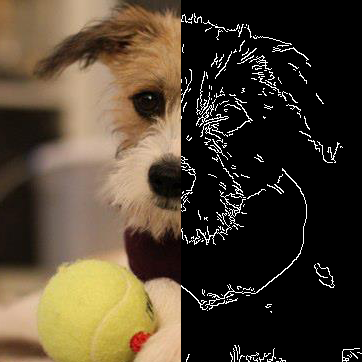
In this image we applied the SimpleCV edges method. This method sets edge pixels in the image to white. Example
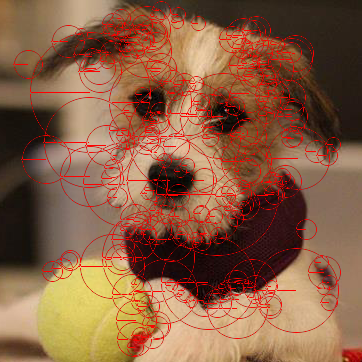
Keypoints are visually unique areas of an image that are used for a variety of 3D reconstruction and image matching tasks. Finding keypoints in SimpleCV is super easy, just call the Image.findKeypoints method. Example